string
c에서 문자열 처리 방법
직관적이지 않기에 c++에서 개선되었다.
<string.h> 혹은 <cstring>은 c언어의 문자열 처리를 위한 헤더이다.
//c에서 문자열 처리하는 방법
#include <stdio.h>
#include <cstring> //<string.h>
int main()
{
char s1[32] = "hello";
const char* s2 = "world";
//s1 = s2; // 대입 : c언어는 배열의 이름을 포인터 상수로 보기 때문에 error 발생
strcpy( s1, s2); // strcpy 함수로 해결 가능
//if ( s1 == s2 ) {} // 비교
if ( strcmp(s1, s2) == 0) {} // strcmp : string compare로 비교 가능
}
c++의 string에서 비교 및 대입
C++에서 String 타입이 새롭게 소개되었다.
<string> 헤더가 포함되어야 한다. std::string 타입 (STL의 string 클래스) 를 사용한다.
문자열관련 연산을 매우 직관적으로 사용할 수 있다.
문자열 처리할 때 string을 사용하면 매우 편리하다.
#include <iostream>
#include <string>
int main()
{
std::string s1 = "hello";
std::string s2 = "world";
std::cout << "1) s1 : " << s1 << ", s2 : " << s2 << std::endl;
s1 = s2; // 대입
std::cout << "2) s1 : " << s1 << ", s2 : " << s2 << std::endl;
if ( s1 == s2 ) // 비교
{
std::cout << "same" << std::endl;
}
std::cout << "3) s1 : " << s1 << ", s2 : " << s2 << std::endl;
std::string s3 = s1 + s2;
std::cout << "4) s3 : " << s3 << std::endl;
}
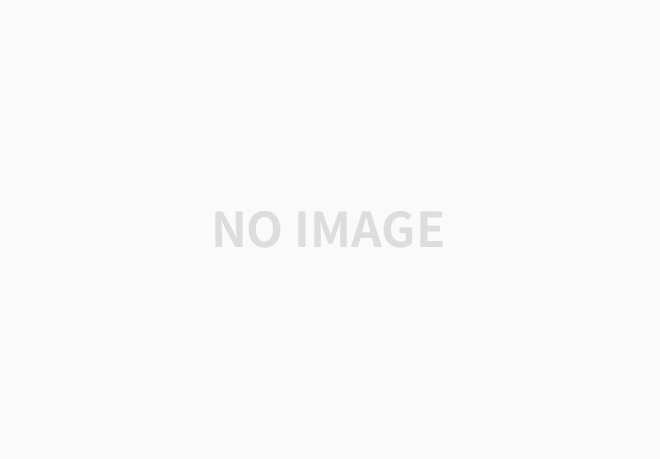
c의 char* 와 c++ string 의 호환 및 처리
// 해당 코드는 c언어에서 문자열을 처리하는 foo 함수이다. c++에서도 사용 가능하다.
// char* 형태의 s 를 받아서 printf로 출력한다.
void foo( const char* s)
{
printf("foo : %s\n", s);
}
std::string s는 c++의 string 형태이기 때문에 foo(s)를 호출하면 error가 발생한다.
(const char*) 를 사용해도 에러가 발생한다.
이 때 s.c_str() 를 활용해서 foo()함수에서 사용이 가능하다.
#include <iostream>
#include <string>
void foo( const char* s)
{
printf("foo : %s\n", s);
}
int main()
{
std::string s = "hello";
foo(s); // error
foo((const char*)s); // error
foo( s.c_str() ); // ok
}
에러 문구:
main.cpp:13:10: error: cannot convert ‘std::string {aka std::basic_string}’ to ‘const char*’ for argument ‘1’ to ‘void foo(const char*)’
main.cpp:14:22: error: invalid cast from type ‘std::string {aka std::basic_string}’ to type ‘const char*’
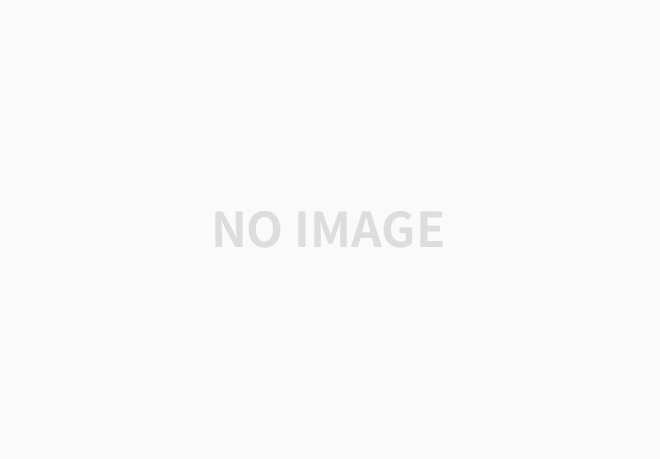
s.c_str() 으로 기존의 char* 형태의 string을 지원한다.
#include <iostream>
#include <string>
void foo( const char* s){
printf("foo : %s\n", s);
}
int main()
{
std::string s = "hello";
foo( s.c_str() ); // ok
}
원하는 형태로 출력이 잘 되는 것을 확인할 수 있다.
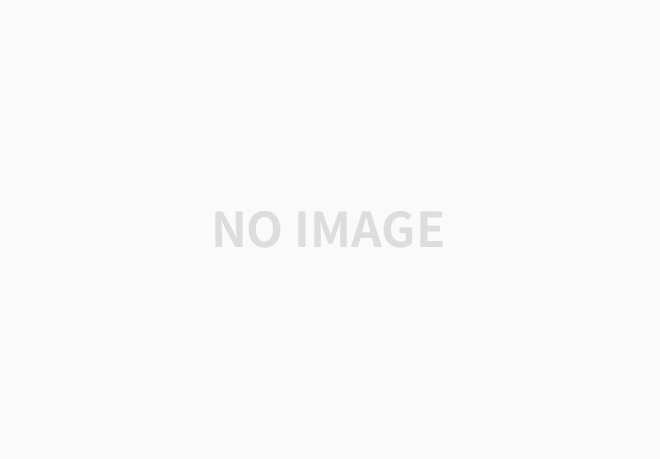
'C++' 카테고리의 다른 글
[C++] 함수 : template, inline (0) | 2021.08.09 |
---|---|
[C++] 함수 : default parameter, function overloading (0) | 2021.08.08 |
[C++] 변수 선언 : constexpr, structure binding (0) | 2021.08.07 |
[C++] 변수 선언 : uniform initialize, auto, decltype, using (0) | 2021.08.06 |
[C++] cout, cin, iomanipulator (0) | 2021.08.05 |
댓글