exception
c언어에서 함수가 실패하면 함수의 반환 값(retrun value)을 사용해서 실패했음을 알린다.
- 반환 값과 실패하는 것이 명확히 분리되지 않는 단점이 있다.
- 정상적인 실행흐름과 오류처리의 코드가 분리되어 있지 않는 단점이 있다.
- 중요하고 심각한 오류라도 오류를 무시할 수 있는 단점이 있다. (오동작에 대한 버그 처리에 어려움)
객체지향 언어들이 사용하는 오류 처리가 바로 exception 처리
- 함수가 실패하면 throw 키워드를 사용해서 예외를 던진다.
- 던져진 예외를 처리하지 않으면 프로그램은 종료된다.
catch문은 여러 개를 만들 수 있다.
catch( ... )은 모든 종류의 예외를 잡을 수 있다. 해당 구문은 맨 나중에만 넣을 수 있다.
#include <iostream>
int readFile()
{
FILE* f = fopen("a.txt", "rt");
if ( f == 0 )
throw "FileNotFound";
//....
fclose(f);
return 0;
}
int main()
{
try
{
int ret = readFile();
//....
}
catch( const char* s)
{
std::cout << s << std::endl;
//exit(0)
}
catch( int n)
{
}
catch( ... )
{
}
std::cout << "main" << std::endl;
}
아래와 같이 FileNotFound 를 출력하는 exception 처리가 수행되었다.
C++ 표준 예외 클래스
이미 C++표준 예외 클래스가 정의되어 있고 이를 사용하는 것이 좋다.
std::exception 에 이미 정의된 것을 사용하면 된다.
exception 전용 클래스
예외 전용 클래스를 만들어서 사용하는 것이 좋다.
- std::exception으로부터 상속 받아서 만드는 것이 좋다.
- 이미 C++표준 예외 클래스가 정의되어 있고 이를 사용하는 것이 좋다.
what() 이라는 가상함수 재정의를 통해서 정보를 출력해볼 수 있다.
#include <iostream>
class FileNotFound : public std::exception
{
public:
virtual const char* what() const noexcept
{
return "File Not Found";
}
};
void foo()
{
throw FileNotFound();
}
int main()
{
try
{
foo();
}
catch(FileNotFound e)
{
std::cout << e.what() << std::endl;
}
}
컴파일러에 따라 출력이 다를 수 있다.
exception catch -> &
exception을 catch 할 때는 참조로 받는다.
catch (std::exception& e)
#include <iostream>
class FileNotFound : public std::exception
{
public:
virtual const char* what() const noexcept
{
return "File Not Found";
}
};
void foo()
{
throw FileNotFound();
}
int main()
{
try
{
foo();
}
catch(std::exception& e)
{
std::cout << e.what() << std::endl;
}
}
exception의 기본에 대해 알아봤습니다.
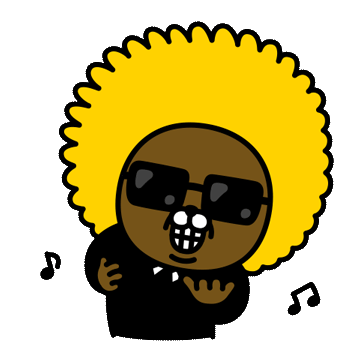
'C++' 카테고리의 다른 글
[C++] 디자인패턴 : protected constructor (0) | 2022.01.27 |
---|---|
[C++] Design Patterns (디자인 패턴) - GoF (0) | 2022.01.26 |
[C++] cout, endl 원리, operator<< 재정의 (0) | 2022.01.02 |
[C++] 연산자 재정의 (operator overloading) (0) | 2022.01.01 |
[C++] 다중 상속 (multiple inheritance) (0) | 2021.12.31 |
댓글